First of all, thanks to Fahim vai, he gave me the idea first...
This is a very easy way to add a cool bookmark item on your browser which can easily locate UVa, SPOJ & TopCoder problems for you. After you follow these steps, you will be able to open any UVa / SPOJ problem with just a click, you even do not need to enter any web address !
UVa Locator:
Right click on bookmark toolbar of your browser.
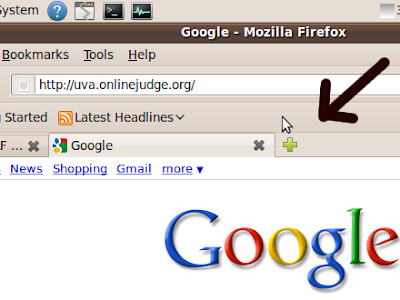
javascript:var%20pid=prompt('Enter%20problem%20number:');
location.href='http://uva.onlinejudge.org/external/'+pid.substring(0,pid.length-2)+'/'+pid+'.html';
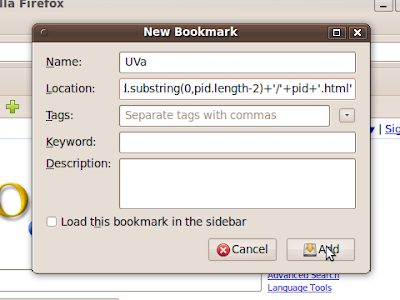
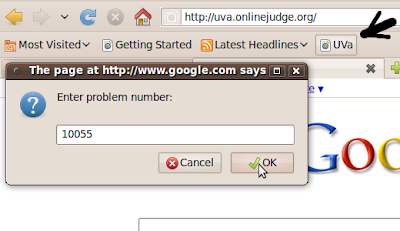
Spoj Locator:
Similar process, add e new bookmark item, now, give a different name, for example, "SPOJ" and on the location, paste the following javascript:
javascript:var%20pid=prompt('Enter%20problem%20name:');
location.href='https://www.spoj.pl/problems/'+pid.toUpperCase()+'/'
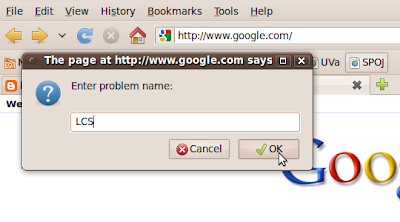
Click "Add" and done!. Now you will get a similar bookmark item as the previous one. But as you know, spoj problems are recognized with their codnames, not numbers. So enter a code name in the textbox, don't bother about case, and click ok. It will open the page of the problem if it is there. For example, to open the problem "Longest Common Substring", it has a codename LCS, you should enter "LCS" in the box, (not necessarily all should be uppercase).
TopCoder Locator:
Similarly, topcoder problems are known by their respective class names, you can add a simple search box for topcoder problems by adding the following javascript in the similar way stated above:
javascript:var%20pid=prompt('Enter%20class%20name:');
location.href='http://www.topcoder.com/tc?module=ProblemArchive&class='+pid
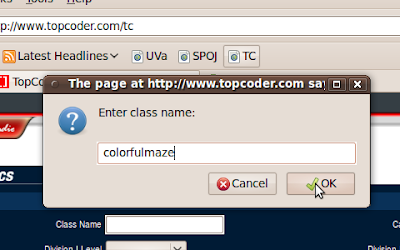
As these will open in current window, make sure you open a new tab before using these to save some back, forward clicks ><><><
Have Fun !!!